Polymorph Type Using Int in Laravel
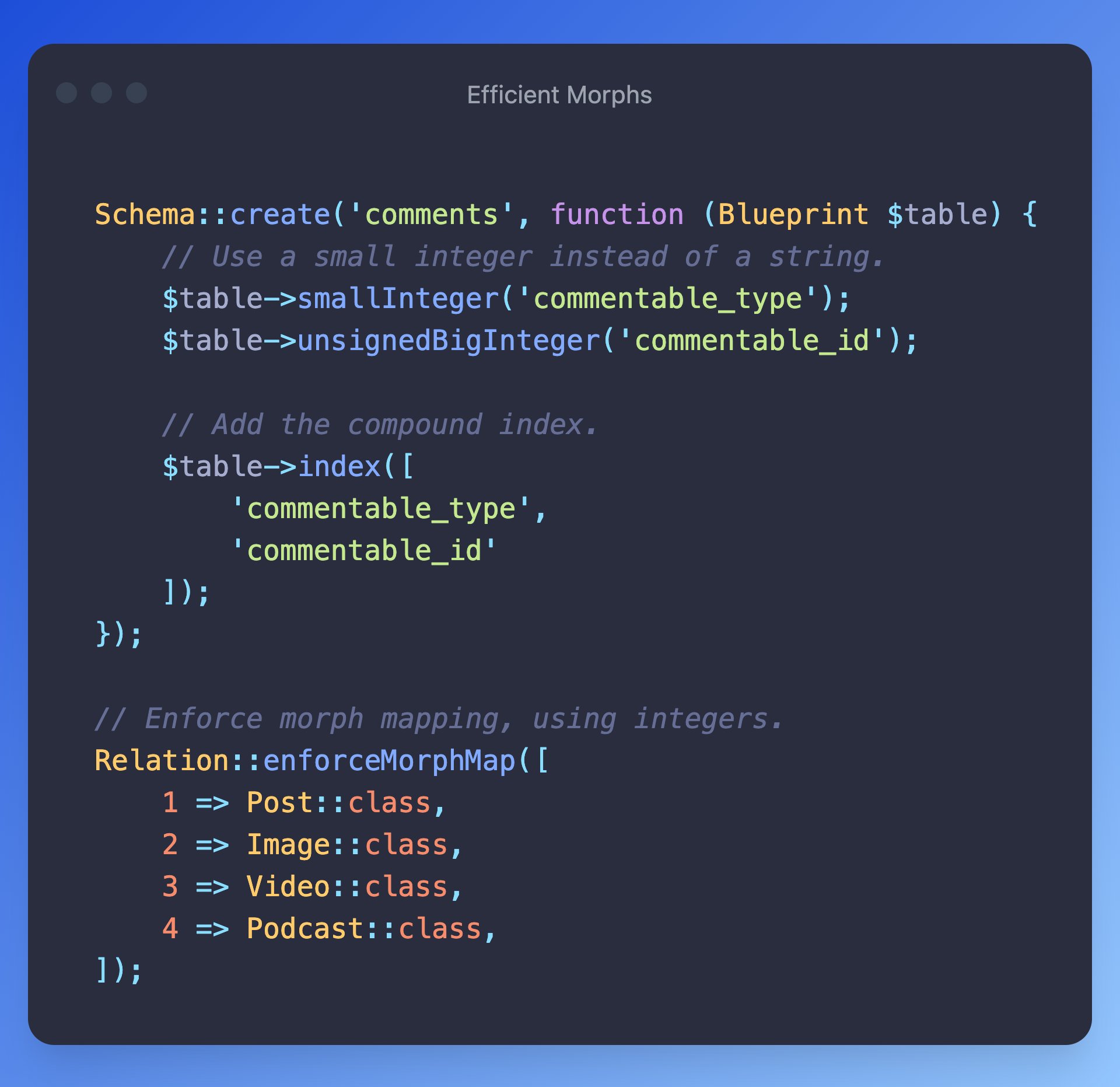
When using polymorphic relationships in Laravel, the type column is typically stored as a string.
However, using string types for indexing can reduce database performance significantly.
As shown in this Twitter thread, using integers instead of strings for polymorphic types can improve query performance.
To implement this, you can map your models to integers in your migrations:
public function up()
{
Schema::create('taggables', function (Blueprint $table) {
$table->id();
$table->unsignedBigInteger('taggable_id');
$table->unsignedTinyInteger('taggable_type'); // Using int instead of string
$table->timestamps();
});
}
And in your model, you can define the mapping:
protected function getMorphClass()
{
return array_search(static::class, [
1 => Post::class,
2 => Video::class,
3 => Photo::class
]);
}